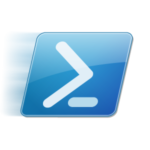
Fill a host file (hosts.txt) with the hostnames you want to retrieve the operating system information
Script :
$Catalog = GC "C:\hosts.Txt" ForEach($Machine in $Catalog){ $QueryString = Gwmi Win32_OperatingSystem -Comp $Machine $QueryString = $QueryString.Caption Write-Host $Machine ":" $QueryString }
Reference
Win32_OperatingSystem class
The Win32_OperatingSystem WMI class represents a Windows-based operating system installed on a computer. Any operating system that can be installed on a computer that can run a Windows-based operating system is a descendent or member of this class. Win32_OperatingSystem is a singleton class. To get the single instance, use “@” for the key.
Windows Server 2003 and Windows XP: If a computer has multiple operating systems installed, this class only returns an instance for the currently active operating system.
The following syntax is simplified from Managed Object Format (MOF) code and includes all of the inherited properties. Properties and methods are in alphabetic order, not MOF order.
Syntax
[Provider("CIMWin32")]class Win32_OperatingSystem : CIM_OperatingSystem { string BootDevice; string BuildNumber; string BuildType; string Caption; string CodeSet; string CountryCode; string CreationClassName; string CSCreationClassName; string CSDVersion; string CSName; sint16 CurrentTimeZone; boolean DataExecutionPrevention_Available; boolean DataExecutionPrevention_32BitApplications; boolean DataExecutionPrevention_Drivers; uint8 DataExecutionPrevention_SupportPolicy; boolean Debug; string Description; boolean Distributed; uint32 EncryptionLevel; uint8 ForegroundApplicationBoost; uint64 FreePhysicalMemory; uint64 FreeSpaceInPagingFiles; uint64 FreeVirtualMemory; datetime InstallDate; uint32 LargeSystemCache; datetime LastBootUpTime; datetime LocalDateTime; string Locale; string Manufacturer; uint32 MaxNumberOfProcesses; uint64 MaxProcessMemorySize; string MUILanguages[]; string Name; uint32 NumberOfLicensedUsers; uint32 NumberOfProcesses; uint32 NumberOfUsers; uint32 OperatingSystemSKU; string Organization; string OSArchitecture; uint32 OSLanguage; uint32 OSProductSuite; uint16 OSType; string OtherTypeDescription; Boolean PAEEnabled; string PlusProductID; string PlusVersionNumber; boolean PortableOperatingSystem; boolean Primary; uint32 ProductType; string RegisteredUser; string SerialNumber; uint16 ServicePackMajorVersion; uint16 ServicePackMinorVersion; uint64 SizeStoredInPagingFiles; string Status; uint32 SuiteMask; string SystemDevice; string SystemDirectory; string SystemDrive; uint64 TotalSwapSpaceSize; uint64 TotalVirtualMemorySize; uint64 TotalVisibleMemorySize; string Version; string WindowsDirectory; };
Properties
The Win32_OperatingSystem class has these properties.
- BootDevice
-
- Data type: string
- Access type: Read-only
Name of the disk drive from which the Windows operating system starts.
Example: “\\Device\Harddisk0”
- BuildNumber
-
- Data type: string
- Access type: Read-only
Build number of an operating system. It can be used for more precise version information than product release version numbers.
Example: “1381”
- BuildType
-
- Data type: string
- Access type: Read-only
Type of build used for an operating system.
Examples: “”retail build””, “”checked build””
- Caption
-
- Data type: string
- Access type: Read-only
Short description of the object—a one-line string. The string includes the operating system version. For example, “Microsoft Windows XP Professional Version = 5.1.2500”. This property can be localized.
- CodeSet
-
- Data type: string
- Access type: Read-only
- Qualifiers: MaxLen (6)
Code page value an operating system uses. A code page contains a character table that an operating system uses to translate strings for different languages. The American National Standards Institute (ANSI) lists values that represent defined code pages. If an operating system does not use an ANSI code page, this member is set to 0 (zero). The CodeSet string can use a maximum of six characters to define the code page value.
Example: “1255”
- CountryCode
-
- Data type: string
- Access type: Read-only
Code for the country/region that an operating system uses. Values are based on international phone dialing prefixes—also referred to as IBM country/region codes. This property can use a maximum of six characters to define the country/region code value.
Example: “1” (United States)
- CreationClassName
-
Name of the first concrete class that appears in the inheritance chain used in the creation of an instance. When used with other key properties of the class, this property allows all instances of this class and its subclasses to be identified uniquely.
- CSCreationClassName
-
- Data type: string
- Access type: Read-only
Creation class name of the scoping computer system.
- CSDVersion
-
- Data type: string
- Access type: Read-only
NULL-terminated string that indicates the latest service pack installed on a computer. If no service pack is installed, the string is NULL.
Example: “Service Pack 3”
- CSName
-
- Data type: string
- Access type: Read-only
Name of the scoping computer system.
- CurrentTimeZone
-
- Data type: sint16
- Access type: Read-only
Number, in minutes, an operating system is offset from Greenwich mean time (GMT). The number is positive, negative, or zero.
- DataExecutionPrevention_32BitApplications
-
- Data type: boolean
- Access type: Read-only
When the data execution prevention hardware feature is available, this property indicates that the feature is set to work for 32-bit applications if True. On 64-bit computers, the data execution prevention feature is configured in the Boot Configuration Data (BCD) store and the properties in Win32_OperatingSystem are set accordingly.
Windows Server 2003 and Windows XP: This property is not available.
- DataExecutionPrevention_Available
-
- Data type: boolean
- Access type: Read-only
Data execution prevention is a hardware feature to prevent buffer overrun attacks by stopping the execution of code on data-type memory pages. If True, then this feature is available. On 64-bit computers, the data execution prevention feature is configured in the BCD store and the properties in Win32_OperatingSystem are set accordingly.
Windows Server 2003 and Windows XP: This property is not available.
- DataExecutionPrevention_Drivers
-
- Data type: boolean
- Access type: Read-only
When the data execution prevention hardware feature is available, this property indicates that the feature is set to work for drivers if True. On 64-bit computers, the data execution prevention feature is configured in the BCD store and the properties in Win32_OperatingSystem are set accordingly.
Windows Server 2003 and Windows XP: This property is not available.
- DataExecutionPrevention_SupportPolicy
-
- Data type: uint8
- Access type: Read-only
Indicates which Data Execution Prevention (DEP) setting is applied. The DEP setting specifies the extent to which DEP applies to 32-bit applications on the system. DEP is always applied to the Windows kernel.
Windows Server 2003 and Windows XP: This property is not available.
The following table lists the possible values.
- Debug
-
- Data type: boolean
- Access type: Read-only
Operating system is a checked (debug) build. If True, the debugging version of User.exe is installed. Checked builds provide error checking, argument verification, and system debugging code. Additional code in a checked binary generates a kernel debugger error message and breaks into the debugger. This helps immediately determine the cause and location of the error. Performance may be affected in a checked build due to the additional code that is executed.
- Description
-
- Data type: string
- Access type: Read/write
Description of the Windows operating system. Some user interfaces for example, those that allow editing of this description, limit its length to 48 characters.
- Distributed
-
- Data type: boolean
- Access type: Read-only
If True, the operating system is distributed across several computer system nodes. If so, these nodes should be grouped as a cluster.
- EncryptionLevel
-
- Data type: uint32
- Access type: Read-only
Windows Server 2003 and Windows XP: Encryption level for secure transactions: 40-bit, 128-bit, or n-bit.
- ForegroundApplicationBoost
-
- Data type: uint8
- Access type: Read/write
Increase in priority is given to the foreground application. Application boost is implemented by giving an application more execution time slices (quantum lengths). A ForegroundApplicationBoost value of 0 (zero) indicates the system boosts the quantum length by 6; if 1, then 12; and if 2 then 18. The default value is 2.
Value Meaning - 0
None - 1
Minimum - 2
(Default) Maximum - FreePhysicalMemory
-
- Data type: uint64
- Access type: Read-only
Number, in kilobytes, of physical memory currently unused and available.
For more information about using uint64 values in scripts, see Scripting in WMI.
- FreeSpaceInPagingFiles
-
- Data type: uint64
- Access type: Read-only
Number, in kilobytes, that can be mapped into the operating system paging files without causing any other pages to be swapped out.
For more information about using uint64 values in scripts, see Scripting in WMI.
- FreeVirtualMemory
-
- Data type: uint64
- Access type: Read-only
Number, in kilobytes, of virtual memory currently unused and available.
For more information about using uint64 values in scripts, see Scripting in WMI.
- InstallDate
-
- Data type: datetime
- Access type: Read-only
Date object was installed. This property does not require a value to indicate that the object is installed.
- LargeSystemCache
-
- Data type: uint32
- Access type: Read-only
Beginning with Windows Vista, this property is obsolete and not supported.
Windows Server 2003 and Windows XP: Indicates whether to optimize memory for applications or system performance.
Value Meaning - 0
Optimize memory for applications. - 1
Optimize memory for system performance. - LastBootUpTime
-
- Data type: datetime
- Access type: Read-only
Date and time the operating system was last restarted.
- LocalDateTime
-
- Data type: datetime
- Access type: Read-only
Operating system version of the local date and time-of-day.
- Locale
-
- Data type: string
- Access type: Read-only
Language identifier used by the operating system. A language identifier is a standard international numeric abbreviation for a country/region. Each language has a unique language identifier (LANGID), a 16-bit value that consists of a primary language identifier and a secondary language identifier.
- Manufacturer
-
- Data type: string
- Access type: Read-only
Name of the operating system manufacturer. For Windows-based systems, this value is “Microsoft Corporation”.
- MaxNumberOfProcesses
-
- Data type: uint32
- Access type: Read-only
Maximum number of process contexts the operating system can support. The default value set by the provider is 4294967295 (0xFFFFFFFF). If there is no fixed maximum, the value should be 0 (zero). On systems that have a fixed maximum, this object can help diagnose failures that occur when the maximum is reached—if unknown, enter 4294967295 (0xFFFFFFFF).
- MaxProcessMemorySize
-
- Data type: uint64
- Access type: Read-only
Maximum number, in kilobytes, of memory that can be allocated to a process. For operating systems with no virtual memory, typically this value is equal to the total amount of physical memory minus the memory used by the BIOS and the operating system. For some operating systems, this value may be infinity, in which case 0 (zero) should be entered. In other cases, this value could be a constant, for example, 2G or 4G.
For more information about using uint64 values in scripts, see Scripting in WMI.
- MUILanguages
-
- Data type: string array
- Access type: Read-only
Multilingual User Interface Pack (MUI Pack ) languages installed on the computer. For example, “en-us”. MUI Pack languages are resource files that can be installed on the English version of the operating system. When an MUI Pack is installed, you can can change the user interface language to one of 33 supported languages.
- Name
-
- Data type: string
- Access type: Read-only
Operating system instance within a computer system.
- NumberOfLicensedUsers
-
- Data type: uint32
- Access type: Read-only
Number of user licenses for the operating system. If unlimited, enter 0 (zero). If unknown, enter -1.
- NumberOfProcesses
-
- Data type: uint32
- Access type: Read-only
Number of process contexts currently loaded or running on the operating system.
- NumberOfUsers
-
- Data type: uint32
- Access type: Read-only
Number of user sessions for which the operating system is storing state information currently.
- OperatingSystemSKU
-
- Data type: uint32
- Access type: Read-only
Stock Keeping Unit (SKU) number for the operating system.
Windows Server 2003 and Windows XP: This property is not available.
The following table lists possible SKU values.
Value Meaning - 0
Undefined - 1
Ultimate Edition - 2
Home Basic Edition - 3
Home Premium Edition - 4
Enterprise Edition - 5
Home Basic N Edition - 6
Business Edition - 7
Standard Server Edition - 8
Datacenter Server Edition - 9
Small Business Server Edition - 10
Enterprise Server Edition - 11
Starter Edition - 12
Datacenter Server Core Edition - 13
Standard Server Core Edition - 14
Enterprise Server Core Edition - 15
Enterprise Server Edition for Itanium-Based Systems - 16
Business N Edition - 17
Web Server Edition - 18
Cluster Server Edition - 19
Home Server Edition - 20
Storage Express Server Edition - 21
Storage Standard Server Edition - 22
Storage Workgroup Server Edition - 23
Storage Enterprise Server Edition - 24
Server For Small Business Edition - 25
Small Business Server Premium Edition - Organization
-
- Data type: string
- Access type: Read-only
Company name for the registered user of the operating system.
Example: “Microsoft Corporation”
- OSArchitecture
-
- Data type: string
- Access type: Read-only
Architecture of the operating system, as opposed to the processor. This property can be localized.
Example: 32-bit
Windows Server 2003 and Windows XP: This property is not available.
- OSLanguage
-
- Data type: uint32
- Access type: Read-only
Language version of the operating system installed. The following table lists the possible values. Example: 0x0807 (German, Switzerland).
Value Meaning - 1 (0x1)
Arabic - 4 (0x4)
Chinese (Simplified)– China - 9 (0x9)
English - 1025 (0x401)
Arabic – Saudi Arabia - 1026 (0x402)
Bulgarian - 1027 (0x403)
Catalan - 1028 (0x404)
Chinese (Traditional) – Taiwan - 1029 (0x405)
Czech - 1030 (0x406)
Danish - 1031 (0x407)
German – Germany - 1032 (0x408)
Greek - 1033 (0x409)
English – United States - 1034 (0x40A)
Spanish – Traditional Sort - 1035 (0x40B)
Finnish - 1036 (0x40C)
French – France - 1037 (0x40D)
Hebrew - 1038 (0x40E)
Hungarian - 1039 (0x40F)
Icelandic - 1040 (0x410)
Italian – Italy - 1041 (0x411)
Japanese - 1042 (0x412)
Korean - 1043 (0x413)
Dutch – Netherlands - 1044 (0x414)
Norwegian – Bokmal - 1045 (0x415)
Polish - 1046 (0x416)
Portuguese – Brazil - 1047 (0x417)
Rhaeto-Romanic - 1048 (0x418)
Romanian - 1049 (0x419)
Russian - 1050 (0x41A)
Croatian - 1051 (0x41B)
Slovak - 1052 (0x41C)
Albanian - 1053 (0x41D)
Swedish - 1054 (0x41E)
Thai - 1055 (0x41F)
Turkish - 1056 (0x420)
Urdu - 1057 (0x421)
Indonesian - 1058 (0x422)
Ukrainian - 1059 (0x423)
Belarusian - 1060 (0x424)
Slovenian - 1061 (0x425)
Estonian - 1062 (0x426)
Latvian - 1063 (0x427)
Lithuanian - 1065 (0x429)
Persian - 1066 (0x42A)
Vietnamese - 1069 (0x42D)
Basque (Basque) - 1070 (0x42E)
Serbian - 1071 (0x42F)
Macedonian (Macedonia (FYROM)) - 1072 (0x430)
Sutu - 1073 (0x431)
Tsonga - 1074 (0x432)
Tswana - 1076 (0x434)
Xhosa - 1077 (0x435)
Zulu - 1078 (0x436)
Afrikaans - 1080 (0x438)
Faeroese - 1081 (0x439)
Hindi - 1082 (0x43A)
Maltese - 1084 (0x43C)
Scottish Gaelic (United Kingdom) - 1085 (0x43D)
Yiddish - 1086 (0x43E)
Malay – Malaysia - 2049 (0x801)
Arabic – Iraq - 2052 (0x804)
Chinese (Simplified) – PRC - 2055 (0x807)
German – Switzerland - 2057 (0x809)
English – United Kingdom - 2058 (0x80A)
Spanish – Mexico - 2060 (0x80C)
French – Belgium - 2064 (0x810)
Italian – Switzerland - 2067 (0x813)
Dutch – Belgium - 2068 (0x814)
Norwegian – Nynorsk - 2070 (0x816)
Portuguese – Portugal - 2072 (0x818)
Romanian – Moldova - 2073 (0x819)
Russian – Moldova - 2074 (0x81A)
Serbian – Latin - 2077 (0x81D)
Swedish – Finland - 3073 (0xC01)
Arabic – Egypt - 3076 (0xC04)
Chinese (Traditional) – Hong Kong SAR - 3079 (0xC07)
German – Austria - 3081 (0xC09)
English – Australia - 3082 (0xC0A)
Spanish – International Sort - 3084 (0xC0C)
French – Canada - 3098 (0xC1A)
Serbian – Cyrillic - 4097 (0x1001)
Arabic – Libya - 4100 (0x1004)
Chinese (Simplified) – Singapore - 4103 (0x1007)
German – Luxembourg - 4105 (0x1009)
English – Canada - 4106 (0x100A)
Spanish – Guatemala - 4108 (0x100C)
French – Switzerland - 5121 (0x1401)
Arabic – Algeria - 5127 (0x1407)
German – Liechtenstein - 5129 (0x1409)
English – New Zealand - 5130 (0x140A)
Spanish – Costa Rica - 5132 (0x140C)
French – Luxembourg - 6145 (0x1801)
Arabic – Morocco - 6153 (0x1809)
English – Ireland - 6154 (0x180A)
Spanish – Panama - 7169 (0x1C01)
Arabic – Tunisia - 7177 (0x1C09)
English – South Africa - 7178 (0x1C0A)
Spanish – Dominican Republic - 8193 (0x2001)
Arabic – Oman - 8201 (0x2009)
English – Jamaica - 8202 (0x200A)
Spanish – Venezuela - 9217 (0x2401)
Arabic – Yemen - 9226 (0x240A)
Spanish – Colombia - 10241 (0x2801)
Arabic – Syria - 10249 (0x2809)
English – Belize - 10250 (0x280A)
Spanish – Peru - 11265 (0x2C01)
Arabic – Jordan - 11273 (0x2C09)
English – Trinidad - 11274 (0x2C0A)
Spanish – Argentina - 12289 (0x3001)
Arabic – Lebanon - 12298 (0x300A)
Spanish – Ecuador - 13313 (0x3401)
Arabic – Kuwait - 13322 (0x340A)
Spanish – Chile - 14337 (0x3801)
Arabic – U.A.E. - 14346 (0x380A)
Spanish – Uruguay - 15361 (0x3C01)
Arabic – Bahrain - 15370 (0x3C0A)
Spanish – Paraguay - 16385 (0x4001)
Arabic – Qatar - 16394 (0x400A)
Spanish – Bolivia - 17418 (0x440A)
Spanish – El Salvador - 18442 (0x480A)
Spanish – Honduras - 19466 (0x4C0A)
Spanish – Nicaragua - 20490 (0x500A)
Spanish – Puerto Rico - OSProductSuite
-
- Data type: uint32
- Access type: Read-only
Installed and licensed system product additions to the operating system. For example, the value of 146 (0x92) for OSProductSuite indicates Enterprise, Terminal Services, and Data Center (bits one, four, and seven set). The following table lists possible values.
Value Meaning - 1 (0x1)
Microsoft Small Business Server was once installed, but may have been upgraded to another version of Windows. Windows Server 2003 and Windows XP: Small Business
- 2 (0x2)
Windows Server 2008 Enterprise or Windows Server 2003, Enterprise Edition is installed. Windows Server 2003 and Windows XP: Enterprise
- 4 (0x4)
Windows BackOffice components are installed. Windows Server 2003 and Windows XP: BackOffice
- 8 (0x8)
Communication Server is installed. Windows Server 2003 and Windows XP: Communication Server
- 16 (0x10)
Terminal Services is installed. Windows Server 2003 and Windows XP: Terminal Services
- 32 (0x20)
Microsoft Small Business Server is installed with the restrictive client license. Windows Server 2003 and Windows XP: Small Business (Restricted)
- 64 (0x40)
Windows XP Embedded is installed. Windows Server 2003 and Windows XP: Embedded NT
- 128 (0x80)
Windows Server 2008 Datacenter, Windows Server 2003, Datacenter Edition, or is installed. Windows Server 2003 and Windows XP: Data Center
- 256 (0x100)
Terminal Services is installed, but only one interactive session is supported. Windows Server 2003 and Windows XP: This value is not available.
- 512 (0x200)
Windows XP Home Edition is installed. Windows Server 2003 and Windows XP: This value is not available.
- 1024 (0x400)
Windows Server 2003, Web Edition is installed. Windows Server 2003 and Windows XP: This value is not available.
- 8192 (0x2000)
Windows Storage Server 2003 R2 is installed. Windows Server 2003 and Windows XP: This value is not available.
- 16384 (0x4000)
Windows Server 2003, Compute Cluster Edition is installed. Windows Server 2003 and Windows XP: This value is not available.
- OSType
-
- Data type: uint16
- Access type: Read-only
Type of operating system. The following list identifies the possible values.
Values Meaning - 0 (0x0)
Unknown - 1 (0x1)
Other - 2 (0x2)
MACROS - 3 (0x3)
ATTUNIX - 4 (0x4)
DGUX - 5 (0x5)
DECNT - 6 (0x6)
Digital UNIX - 7 (0x7)
OpenVMS - 8 (0x8)
HPUX - 9 (0x9)
AIX - 10 (0xA)
MVS - 11 (0xB)
OS400 - 12 (0xC)
OS/2 - 13 (0xD)
JavaVM - 14 (0xE)
MSDOS - 15 (0xF)
WIN3x - 16 (0x10)
WIN95 - 17 (0x11)
WIN98 - 18 (0x12)
WINNT - 19 (0x13)
WINCE - 20 (0x14)
NCR3000 - 21 (0x15)
NetWare - 22 (0x16)
OSF - 23 (0x17)
DC/OS - 24 (0x18)
Reliant UNIX - 25 (0x19)
SCO UnixWare - 26 (0x1A)
SCO OpenServer - 27 (0x1B)
Sequent - 28 (0x1C)
IRIX - 29 (0x1D)
Solaris - 30 (0x1E)
SunOS - 31 (0x1F)
U6000 - 32 (0x20)
ASERIES - 33 (0x21)
TandemNSK - 34 (0x22)
TandemNT - 35 (0x23)
BS2000 - 36 (0x24)
LINUX - 37 (0x25)
Lynx - 38 (0x26)
XENIX - 39 (0x27)
VM/ESA - 40 (0x28)
Interactive UNIX - 41 (0x29)
BSDUNIX - 42 (0x2A)
FreeBSD - 43 (0x2B)
NetBSD - 44 (0x2C)
GNU Hurd - 45 (0x2D)
OS9 - 46 (0x2E)
MACH Kernel - 47 (0x2F)
Inferno - 48 (0x30)
QNX - 49 (0x31)
EPOC - 50 (0x32)
IxWorks - 51 (0x33)
VxWorks - 52 (0x34)
MiNT - 53 (0x35)
BeOS - 54 (0x36)
HP MPE - 55 (0x37)
NextStep - 56 (0x38)
PalmPilot - 57 (0x39)
Rhapsody - OtherTypeDescription
-
- Data type: string
- Access type: Read-only
Additional description for the current operating system version.
Windows Server 2003 R2: Contains the string “R2”.
Windows Server 2003 and Windows XP: OtherTypeDescription is null.
- PAEEnabled
-
- Data type: Boolean
- Access type: Read-only
If True, the physical address extensions (PAE) are enabled by the operating system running on Intel processors. PAE allows applications to address more than 4 GB of physical memory. When PAE is enabled, the operating system uses three-level linear address translation rather than two-level. Providing more physical memory to an application reduces the need to swap memory to the page file and increases performance. To enable, PAE, use the “/PAE” switch in the Boot.ini file. For more information about the Physical Address Extension feature, see http://go.microsoft.com/fwlink?LinkID=45912.
Windows XP: The PAEEnabled property is not available.
- PlusProductID
-
- Data type: string
- Access type: Read-only
Identification number for the Windows Plus! operating system enhancement software—if installed.
- PlusVersionNumber
-
- Data type: string
- Access type: Read-only
Version number of the Windows Plus! operating system enhancement software—if installed.
- PortableOperatingSystem
-
- Data type: boolean
- Access type: Read-only
Specifies whether the operating system booted from an external USB device. If true, the operating system has detected it is booting on a supported locally connected storage device.
- Primary
-
- Data type: boolean
- Access type: Read-only
Specifies whether this is the primary operating system.
- ProductType
-
- Data type: uint32
- Access type: Read-only
Additional system information.
Value Meaning - 1
Work Station - 2
Domain Controller - 3
Server - RegisteredUser
-
- Data type: string
- Access type: Read-only
Name of the registered user of the operating system.
Example: “Ben Smith”
- SerialNumber
-
- Data type: string
- Access type: Read-only
Operating system product serial identification number.
Example: “10497-OEM-0031416-71674”
- ServicePackMajorVersion
-
- Data type: uint16
- Access type: Read-only
Major version number of the service pack installed on the computer system. If no service pack has been installed, the value is 0 (zero).
- ServicePackMinorVersion
-
- Data type: uint16
- Access type: Read-only
Minor version number of the service pack installed on the computer system. If no service pack has been installed, the value is 0 (zero).
- SizeStoredInPagingFiles
-
- Data type: uint64
- Access type: Read-only
Total number of kilobytes that can be stored in the operating system paging files—0 (zero) indicates that there are no paging files. Be aware that this number does not represent the actual physical size of the paging file on disk.
For more information about using uint64 values in scripts, see Scripting in WMI.
- Status
-
- Data type: string
- Access type: Read-only
Current status of the object. Various operational and nonoperational statuses can be defined. Operational statuses include: “OK”, “Degraded”, and “Pred Fail” (an element, such as a SMART-enabled hard disk drive may function properly, but predicts a failure in the near future). Nonoperational statuses include: “Error”, “Starting”, “Stopping”, and “Service”. The Service status applies to administrative work, such as mirror-resilvering of a disk, reload of a user permissions list, or other administrative work. Not all such work is online, but the managed element is neither “OK” nor in one of the other states.
The values are:
- “OK”
- “Error”
- “Degraded”
- “Unknown”
- “Pred Fail”
- “Starting”
- “Stopping”
- “Service”
- SuiteMask
-
- Data type: uint32
- Access type: Read-only
Bit flags that identify the product suites available on the system.
For example, to specify both Personal and BackOffice, set SuiteMask to 4 | 512 or 516.
The following table lists possible values.
Value Meaning - 1
Small Business - 2
Enterprise - 4
BackOffice - 8
Communications - 16
Terminal - 32
Small Business Restricted - 64
Embedded NT - 128
Data Center - 256
Single User - 512
Personal - 1024
Blade - SystemDevice
-
- Data type: string
- Access type: Read-only
Physical disk partition on which the operating system is installed.
- SystemDirectory
-
- Data type: string
- Access type: Read-only
System directory of the operating system.
Example: “C:\WINDOWS\SYSTEM32”
- SystemDrive
-
- Data type: string
- Access type: Read-only
Letter of the disk drive on which the operating system resides. Example: “C:”
- TotalSwapSpaceSize
-
- Data type: uint64
- Access type: Read-only
Total swap space in kilobytes. This value may be NULL (unspecified) if the swap space is not distinguished from page files. However, some operating systems distinguish these concepts. For example, in UNIX, whole processes can be swapped out when the free page list falls and remains below a specified amount.
For more information about using uint64 values in scripts, see Scripting in WMI.
- TotalVirtualMemorySize
-
- Data type: uint64
- Access type: Read-only
Number, in kilobytes, of virtual memory. For example, this may be calculated by adding the amount of total RAM to the amount of paging space, that is, adding the amount of memory in or aggregated by the computer system to the property, SizeStoredInPagingFiles.
For more information about using uint64 values in scripts, see Scripting in WMI.
- TotalVisibleMemorySize
-
- Data type: uint64
- Access type: Read-only
Total amount, in kilobytes, of physical memory available to the operating system. This value does not necessarily indicate the true amount of physical memory, but what is reported to the operating system as available to it.
For more information about using uint64 values in scripts, see Scripting in WMI.
- Version
-
- Data type: string
- Access type: Read-only
Version number of the operating system.
Example: “4.0”
- WindowsDirectory
-
- Data type: string
- Access type: Read-only
Windows directory of the operating system.
Example: “C:\WINDOWS”