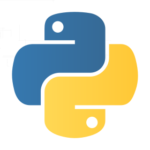
This script can be useful if you want to create a web picture gallery. The first version is described here. This version is using Python and the ImageMagick tool convert (documentation here).
The script will resize pictures in two formats :
- thumbnail : square size 200px
- web “full size” : 1080px
You will have to set the following variable with your own folder settings :
- thumbnail folder : dest_thumb
- web “full size” folder : dest_resize
- source image folder : source
The folder structure is automatically created in both destination folders (thumbnail and web folders). Comments are welcome !
#!/usr/bin/env python # -*- coding: utf8 -*- import os import re import collections import subprocess import shutil import PIL from PIL import Image from glob import glob dest_thumb="/images/thumbnail/" param_thumb=['-thumbnail', 'x200', '-resize', '200x<', '-resize', '50%', '-gravity', 'center', '-crop', '100x100+0+0', '+repage', '-format', 'jpg', '-quality', '91', '-auto-orient'] dest_resize="/images/resized/" param_resize =['-geometry', 'x1080', '-auto-orient'] source="/images/full/" convert_tool="/usr/bin/convert" result = dict() for root, dirs, files in os.walk(source, topdown=True): for name in files: if re.search(r'\.jpeg$|\.jpg$', name, re.IGNORECASE): result.setdefault(os.path.relpath(root, source),[]).append(name) dest_folder_list = { dest_thumb: param_thumb, dest_resize: param_resize } for destfoldername, param_resize in dest_folder_list.items(): # CONVERT for key in result: if not (os.path.isdir(destfoldername+key)): os.makedirs(destfoldername+key) for filename in result[key]: # REMOVE HIDDEN FILE THAT BEGIN WITH "._" if re.search(r'(^\.\_).*(\.jpeg$|\.jpg$)', filename, re.IGNORECASE): os.remove(source+key+"/"+filename) else: if not (os.path.exists(destfoldername+key+"/"+filename)): srcfile = source+key+"/"+filename dstfile = destfoldername+key+"/"+filename cnv_cmd = [ convert_tool , srcfile ] + param_resize + [ dstfile ] p = subprocess.Popen(cnv_cmd) # CLEAN THUMB AND RESIZE FOLDER result_clean = dict() for root_clean, dirs_clean, files_clean in os.walk(destfoldername, topdown=True): for name_clean in files_clean: if re.search(r'\.jpeg$|\.jpg$', name_clean, re.IGNORECASE): result_clean.setdefault(os.path.relpath(root_clean, destfoldername),[]).append(name_clean) for key in result_clean: if not (os.path.exists(source+key)): shutil.rmtree(destfoldername+key,ignore_errors=True)
My Powershell script categories
- Active Directory
- Cluster
- Database
- Exchange
- Files and folders
- Hardware
- Network
- Operating System
- PKI
- SCCM
- Service and process
- Tips
- VMWare
Automatic pictures resizing with Imagemagick