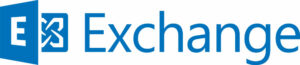
This simple script to check if an email address is the primary one defined in Exchange. For that case, the attribute WindowsEmailAddress is compared to the provided email in the variable $email .
# Exchange PS module is required to run this script # email address to test $email = "myname@domain.net" # Test if tested email is the primary email. If not, exit if ((Get-Mailbox $email).WindowsEmailAddress -notmatch $email) { throw "-- ERROR: not the Primary email --" } else { (Get-Mailbox $email) | select DistinguishedName }
My Powershell script categories
- Active Directory
- Cluster
- Database
- Exchange
- Files and folders
- Hardware
- Network
- Operating System
- PKI
- SCCM
- Service and process
- Tips
- VMWare
Reference
Syntax
Get-Mailbox [-Identity]
Get-Mailbox [-MailboxPlan]
Get-Mailbox [-Server]
Get-Mailbox [-Database]
Get-Mailbox [-Anr]
COMMON PARAMETERS: [-Arbitration] [-Archive ] [-AuditLog ] [-Credential ] [-DomainController ] [-Filter ] [-GroupMailbox ] [-IgnoreDefaultScope ] [-InactiveMailboxOnly ] [-IncludeInactiveMailbox ] [-Monitoring ] [-OrganizationalUnit ] [-PublicFolder ] [-ReadFromDomainController ] [-RecipientTypeDetails ] [-RemoteArchive ] [-ResultSize ] [-SoftDeletedMailbox ] [-SortBy ]
Examples
Example 1
This example returns a summary list of all the mailboxes in your organization.
Get-Mailbox -ResultSize unlimited
Example 2
This example returns a list of all the mailboxes in your organization in the Users OU.
Get-Mailbox -OrganizationalUnit Users
Example 3
This example returns all the mailboxes that resolve from the ambiguous name resolution search on the string “Chr”. This example returns mailboxes for users such as Chris Ashton, Christian Hess, and Christa Geller.
Get-Mailbox -Anr Chr
Example 4
This example returns a summary list of all archive mailboxes on the Mailbox server named Mailbox01.
Get-Mailbox -Archive -Server Mailbox01
Example 5
This example returns information about the remote archive mailbox for the user ed@contoso.com.
Get-Mailbox -Identity ed@contoso.com -RemoteArchive
Detailed Description
![]() |
---|
When you use the Get-Mailbox cmdlet in on-premises Exchange environments to view the quota settings for a mailbox, you first need to check the value of the UseDatabaseQuotaDefaults property. The value True means per-mailbox quota settings are ignored, and you need to use the Get-MailboxDatabase cmdlet to see the actual values. If the UseDatabaseQuotaDefaults property is False, the per-mailbox quota settings are used, so what you see with the Get-Mailbox cmdlet are the actual quota values for the mailbox. |
You need to be assigned permissions before you can run this cmdlet. Although all parameters for this cmdlet are listed in this topic, you may not have access to some parameters if they’re not included in the permissions assigned to you. To see what permissions you need, see the “Recipient Provisioning Permissions” section in the Recipients Permissions topic.
Parameters
Parameter | Required | Type | Description | ||
---|---|---|---|---|---|
Anr | Optional | System.String | The Anr parameter specifies a string on which to perform an ambiguous name resolution (ANR) search. You can specify a partial string and search for objects with an attribute that matches that string. The default attributes searched are:
|
||
Arbitration | Optional | System.Management.Automation.SwitchParameter | This parameter is available only in on-premises Exchange 2016.
The Arbitration parameter specifies that the mailbox for which you are executing the command is an arbitration mailbox. Arbitration mailboxes are used for managing approval workflow. For example, an arbitration mailbox is used for handling moderated recipients and distribution group membership approval. |
||
Archive | Optional | System.Management.Automation.SwitchParameter | The Archive switch filters the results by archive mailboxes. When you use this switch, only archive mailboxes are included in the results. This switch is required to return archive mailboxes. You don’t need to specify a value with this switch. | ||
AuditLog | Optional | System.Management.Automation.SwitchParameter | This parameter is reserved for internal Microsoft use. | ||
Credential | Optional | System.Management.Automation.PSCredential | This parameter is available only in on-premises Exchange 2016.
The Credential parameter specifies the user name and password that’s used to run this command. Typically, you use this parameter in scripts or when you need to provide different credentials that have the required permissions. This parameter requires the creation and passing of a credential object. This credential object is created by using the Get-Credential cmdlet. For more information, see Get-Credential. |
||
Database | Optional | Microsoft.Exchange.Configuration.Tasks.DatabaseIdParameter | This parameter is available only in on-premises Exchange 2016.
The Database parameter filters the results by mailbox database. When you use this parameter, only mailboxes on the specified database are included in the results. You can any value that uniquely identifies the database. For example:
You can’t use this parameter with the Anr, Identity, or Server parameters. |
||
DomainController | Optional | Microsoft.Exchange.Data.Fqdn | This parameter is available only in on-premises Exchange 2016.
The DomainController parameter specifies the domain controller that’s used by this cmdlet to read data from or write data to Active Directory. You identify the domain controller by its fully qualified domain name (FQDN). For example, dc01.contoso.com. |
||
Filter | Optional | System.String | The Filter parameter indicates the OPath filter used to filter recipients.
For more information about the filterable properties, see Filterable properties for the -Filter parameter. |
||
GroupMailbox | Optional | System.Management.Automation.SwitchParameter | The GroupMailbox switch filters the results by Office 365 groups. When you use this switch, Office 365 groups are included in the results. This switch is required to return Office 365 groups. You don’t need to specify a value with this switch. | ||
Identity | Optional | Microsoft.Exchange.Configuration.Tasks.MailboxIdParameter | The Identity parameter specifies the mailbox that you want to view. You can use any value that uniquely identifies the mailbox.
For example:
You can’t use this parameter with the Anr, Database, MailboxPlan or Server parameters. |
||
IgnoreDefaultScope | Optional | System.Management.Automation.SwitchParameter | This parameter is available only in on-premises Exchange 2016.
The IgnoreDefaultScope switch tells the command to ignore the default recipient scope setting for the Exchange Management Shell session, and to use the entire forest as the scope. This allows the command to access Active Directory objects that aren’t currently available in the default scope. Using the IgnoreDefaultScope switch introduces the following restrictions:
|
||
InactiveMailboxOnly | Optional | System.Management.Automation.SwitchParameter | This parameter is available only in the cloud-based service.
The InactiveMailboxOnly switch filters the results by inactive mailboxes. When you use this switch, only inactive mailboxes are included in the results. You don’t need to specify a value with this switch. An inactive mailbox is a mailbox that’s placed on Litigation Hold or In-Place Hold before it’s soft-deleted. The contents of an inactive mailbox are preserved until the hold is removed. |
||
IncludeInactiveMailbox | Optional | System.Management.Automation.SwitchParameter | This parameter is available only in the cloud-based service.
The IncludeInactiveMailboxswitch specifies that the command returns both active and inactive mailboxes. You don’t need to specify a value with this switch. An inactive mailbox is a mailbox that’s placed on Litigation Hold or In-Place Hold before it’s soft-deleted. The contents of an inactive mailbox are preserved until the hold is removed. |
||
MailboxPlan | Optional | Microsoft.Exchange.Configuration.Tasks.MailboxPlanIdParameter | This parameter is available only in the cloud-based service.
The MailboxPlan parameter filters the results by mailbox plan. When you use this parameter, only mailboxes that are assigned the specified mailbox plan are returned in the results. You can use any value that uniquely identifies the mailbox plan. For example:
A mailbox plan specifies the permissions and features available to a mailbox user in cloud-based organizations. You can see the available mailbox plans by using the Get-MailboxPlancmdlet. You can’t use this parameter with the Anr or Identity parameters. |
||
Monitoring | Optional | System.Management.Automation.SwitchParameter | This parameter is available only in on-premises Exchange 2016.
The Monitoringswitchfilters the results by monitoring mailboxes. When you use this switch, only monitoring mailboxes are included in the results. This switch is required to return monitoring mailboxes. You don’t need to specify a value with this switch. Monitoring mailboxes are associated with managed availability and the Exchange Health Manager service, and have a RecipientTypeDetails property value of MonitoringMailbox. |
||
OrganizationalUnit | Optional | Microsoft.Exchange.Configuration.Tasks.OrganizationalUnitIdParameter | The OrganizationalUnit parameter filters the results based on the object’s location in Active Directory. Only objects that exist in the specified location are returned. Valid input for this parameter is an organizational unit (OU) or domain that’s visible using the Get-OrganizationalUnit cmdlet. You can use any value that uniquely identifies the OU or domain. For example:
|
||
PublicFolder | Optional | System.Management.Automation.SwitchParameter | The PublicFolder switch filters the results by public folder mailboxes. When you use this switch, only public folder mailboxes are included in the results. This switch is required to return public folder mailboxes. You don’t need to specify a value with this switch. | ||
ReadFromDomainController | Optional | System.Management.Automation.SwitchParameter | This parameter is available only in on-premises Exchange 2016.
The ReadFromDomainController switch specifies that information should be read from a domain controller in the user’s domain. If you run the command Set-AdServerSettings -ViewEntireForest $true to include all objects in the forest and you don’t use the ReadFromDomainController switch, it’s possible that information will be read from a global catalog that has outdated information. When you use the ReadFromDomainController switch, multiple reads might be necessary to get the information. You don’t have to specify a value with this switch.
|
||
RecipientTypeDetails | Optional | Microsoft.Exchange.Data.Directory.Recipient.RecipientTypeDetails[] | The RecipientTypeDetails parameter specifies the type of recipients returned. Recipient types are divided into recipient types and subtypes. Each recipient type contains all common properties for all subtypes. For example, the type UserMailbox represents a user account in Active Directory that has an associated mailbox. Because there are several mailbox types, each mailbox type is identified by the RecipientTypeDetails parameter. For example, a conference room mailbox has RecipientTypeDetails set to ConferenceRoomMailbox, whereas a user mailbox has RecipientTypeDetails set to UserMailbox.
You can select from the following values:
|
||
RemoteArchive | Optional | System.Management.Automation.SwitchParameter | This parameter is available only in on-premises Exchange 2016.
The RemoteArchiveswitch filters the results by remote archive mailboxes. When you use this switch, only remote archive mailboxes are included in the results. This switch is required to return remote archive mailboxes. You don’t need to specify a value with this switch. Remote archive mailboxes are archive mailboxes in the cloud-based service that are associated with mailbox users in on-premises Exchange organizations. |
||
ResultSize | Optional | Microsoft.Exchange.Data.Unlimited | The ResultSize parameter specifies the maximum number of results to return. If you want to return all requests that match the query, use unlimited for the value of this parameter. The default value is 1000. | ||
Server | Optional | Microsoft.Exchange.Configuration.Tasks.ServerIdParameter | This parameter is available only in on-premises Exchange 2016.
The Server parameter filters the results by Exchange server. When you use this parameter, only mailboxes on the specified Exchange server are included in the results. You can use any value that uniquely identifies the server. For example:
You can’t use this parameter with the Anr, Database, or Identity parameters.
|
||
SoftDeletedMailbox | Optional | System.Management.Automation.SwitchParameter | This parameter is available only in the cloud-based service.
The SoftDeletedMailbox switch filters the results by soft-deleted mailboxes. When you use this switch, only soft-deleted mailboxes are included in the results. This switch is required to return soft-deleted mailboxes. You don’t need to specify a value with this switch. Soft-deleted mailboxes are deleted mailboxes that are still recoverable. |
||
SortBy | Optional | System.String | The SortBy parameter specifies the property to sort the results by. You can sort by only one property at a time. The results are sorted in ascending order.
If the default view doesn’t include the property you’re sorting by, you can append the command with | Format-Table -Auto You can sort by the following properties:
|
||
AccountPartition | Optional | Microsoft.Exchange.Configuration.Tasks.AccountPartitionIdParameter | This parameter is reserved for internal Microsoft use. | ||
Organization | Optional | Microsoft.Exchange.Configuration.Tasks.OrganizationIdParameter | This parameter is reserved for internal Microsoft use. |