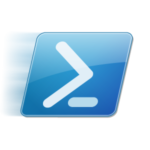
With the following powershell script, you can get a service status on remote servers
Script :
$ErrorActionPreference = "SilentlyContinue" $strCategory = "computer" $objDomain = New-Object System.DirectoryServices.DirectoryEntry $objSearcher = New-Object System.DirectoryServices.DirectorySearcher $objSearcher.SearchRoot = $objDomain $objSearcher.Filter = ("(objectCategory=$strCategory)") $colProplist = "name" , "operatingsystem" foreach ($i in $colPropList){$objSearcher.PropertiesToLoad.Add($i)} $colResults = $objSearcher.FindAll() foreach ($objResult in $colResults) { $objComputer = $objResult.Properties if ($objComputer.operatingsystem -like "*Server*") { Write-Host $objComputer.name ":" $objComputer.operatingsystem Get-Service -ComputerName $objComputer.name | where {$_.Name -match "CcmExec"} } }
References
ServiceController Class
Represents a Windows service and allows you to connect to a running or stopped service, manipulate it, or get information about it.
Namespace: System.ServiceProcess
Assembly: System.ServiceProcess (in System.ServiceProcess.dll)
The following example demonstrates the use of the ServiceController class to control the SimpleService service example.
Imports System Imports System.ServiceProcess Imports System.Diagnostics Imports System.Threading Class Program Public Enum SimpleServiceCustomCommands StopWorker = 128 RestartWorker CheckWorker End Enum 'SimpleServiceCustomCommands Shared Sub Main(ByVal args() As String) Dim scServices() As ServiceController scServices = ServiceController.GetServices() Dim scTemp As ServiceController For Each scTemp In scServices If scTemp.ServiceName = "Simple Service" Then ' Display properties for the Simple Service sample ' from the ServiceBase example Dim sc As New ServiceController("Simple Service") Console.WriteLine("Status = " + sc.Status.ToString()) Console.WriteLine("Can Pause and Continue = " + _ sc.CanPauseAndContinue.ToString()) Console.WriteLine("Can ShutDown = " + sc.CanShutdown.ToString()) Console.WriteLine("Can Stop = " + sc.CanStop.ToString()) If sc.Status = ServiceControllerStatus.Stopped Then sc.Start() While sc.Status = ServiceControllerStatus.Stopped Thread.Sleep(1000) sc.Refresh() End While End If ' Issue custom commands to the service ' enum SimpleServiceCustomCommands ' { StopWorker = 128, RestartWorker, CheckWorker }; sc.ExecuteCommand(Fix(SimpleServiceCustomCommands.StopWorker)) sc.ExecuteCommand(Fix(SimpleServiceCustomCommands.RestartWorker)) sc.Pause() While sc.Status <> ServiceControllerStatus.Paused Thread.Sleep(1000) sc.Refresh() End While Console.WriteLine("Status = " + sc.Status.ToString()) sc.Continue() While sc.Status = ServiceControllerStatus.Paused Thread.Sleep(1000) sc.Refresh() End While Console.WriteLine("Status = " + sc.Status.ToString()) sc.Stop() While sc.Status <> ServiceControllerStatus.Stopped Thread.Sleep(1000) sc.Refresh() End While Console.WriteLine("Status = " + sc.Status.ToString()) Dim argArray() As String = {"ServiceController arg1", "ServiceController arg2"} sc.Start(argArray) While sc.Status = ServiceControllerStatus.Stopped Thread.Sleep(1000) sc.Refresh() End While Console.WriteLine("Status = " + sc.Status.ToString()) ' Display the event log entries for the custom commands ' and the start arguments. Dim el As New EventLog("Application") Dim elec As EventLogEntryCollection = el.Entries Dim ele As EventLogEntry For Each ele In elec If ele.Source.IndexOf("SimpleService.OnCustomCommand") >= 0 Or ele.Source.IndexOf("SimpleService.Arguments") >= 0 Then Console.WriteLine(ele.Message) End If Next ele End If Next scTemp End Sub 'Main End Class 'Program ' This sample displays the following output if the Simple Service ' sample is running: 'Status = Running 'Can Pause and Continue = True 'Can ShutDown = True 'Can Stop = True 'Status = Paused 'Status = Running 'Status = Stopped 'Status = Running '4:14:49 PM - Custom command received: 128 '4:14:49 PM - Custom command received: 129 'ServiceController arg1 'ServiceController arg2 using System; using System.ServiceProcess; using System.Diagnostics; using System.Threading; namespace ServiceControllerSample { class Program { public enum SimpleServiceCustomCommands { StopWorker = 128, RestartWorker, CheckWorker }; static void Main(string[] args) { ServiceController[] scServices; scServices = ServiceController.GetServices(); foreach (ServiceController scTemp in scServices) { if (scTemp.ServiceName == "Simple Service") { // Display properties for the Simple Service sample // from the ServiceBase example. ServiceController sc = new ServiceController("Simple Service"); Console.WriteLine("Status = " + sc.Status); Console.WriteLine("Can Pause and Continue = " + sc.CanPauseAndContinue); Console.WriteLine("Can ShutDown = " + sc.CanShutdown); Console.WriteLine("Can Stop = " + sc.CanStop); if (sc.Status == ServiceControllerStatus.Stopped) { sc.Start(); while (sc.Status == ServiceControllerStatus.Stopped) { Thread.Sleep(1000); sc.Refresh(); } } // Issue custom commands to the service // enum SimpleServiceCustomCommands // { StopWorker = 128, RestartWorker, CheckWorker }; sc.ExecuteCommand((int)SimpleServiceCustomCommands.StopWorker); sc.ExecuteCommand((int)SimpleServiceCustomCommands.RestartWorker); sc.Pause(); while (sc.Status != ServiceControllerStatus.Paused) { Thread.Sleep(1000); sc.Refresh(); } Console.WriteLine("Status = " + sc.Status); sc.Continue(); while (sc.Status == ServiceControllerStatus.Paused) { Thread.Sleep(1000); sc.Refresh(); } Console.WriteLine("Status = " + sc.Status); sc.Stop(); while (sc.Status != ServiceControllerStatus.Stopped) { Thread.Sleep(1000); sc.Refresh(); } Console.WriteLine("Status = " + sc.Status); String[] argArray = new string[] { "ServiceController arg1", "ServiceController arg2" }; sc.Start(argArray); while (sc.Status == ServiceControllerStatus.Stopped) { Thread.Sleep(1000); sc.Refresh(); } Console.WriteLine("Status = " + sc.Status); // Display the event log entries for the custom commands // and the start arguments. EventLog el = new EventLog("Application"); EventLogEntryCollection elec = el.Entries; foreach (EventLogEntry ele in elec) { if (ele.Source.IndexOf("SimpleService.OnCustomCommand") >= 0 | ele.Source.IndexOf("SimpleService.Arguments") >= 0) Console.WriteLine(ele.Message); } } } } } } // This sample displays the following output if the Simple Service // sample is running: //Status = Running //Can Pause and Continue = True //Can ShutDown = True //Can Stop = True //Status = Paused //Status = Running //Status = Stopped //Status = Running //4:14:49 PM - Custom command received: 128 //4:14:49 PM - Custom command received: 129 //ServiceController arg1 //ServiceController arg2
Get a service status on remote servers